Sessions
General Information
A session is a file that's stored on the web server. Not the browser.
A session file can hold more data than a cookie.
When a session is started a cookie is sent to the user that references the session file.
Sessions conceal data values unlike cookies.
Sessions expire when the browser is closed.
Example
To begin a session you just say session start. Sessions use cookies and cookies use headers. A header should come before any html ouput (this is good practice). So that means starting a session would take place before any html output.
<?php session_start(); ?> <!DOCTYPE html PUBLIC> <html lang="en"> <head> <title>Sessions</title> </head> <body> </body> </html>
Once you start a session, a cookie is created. If working locally you can view this cookie by going into your browser preferences. Once you locate where you can view cookies, one should be named by PHPSESSID from your localhost.
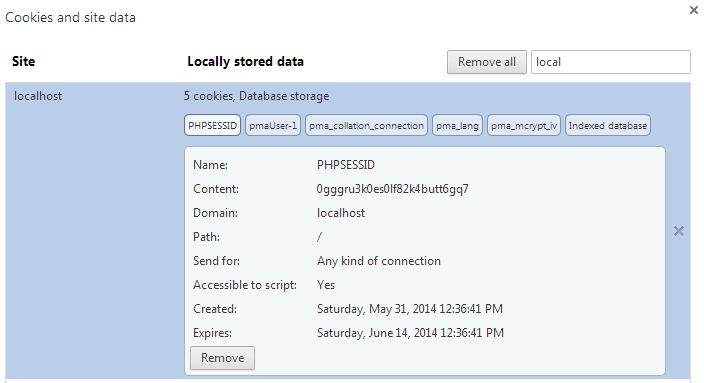
To begin putting data into a session file you just use the super global $_SESSION. The following would echo your first name using a session.
<?php session_start(); ?> <!DOCTYPE html PUBLIC> <html lang="en"> <head> <title>Sessions</title> </head> <body> <?php $_SESSION["first_name"] = "Your name here"; $name = $_SESSION["first_name"]; echo $name; ?> </body> </html>
Uses
Sessions are great for storing information on the web server for later use. Some examples of this kind of information includes shopping carts, logging a user in and out, displaying messages, etc.